
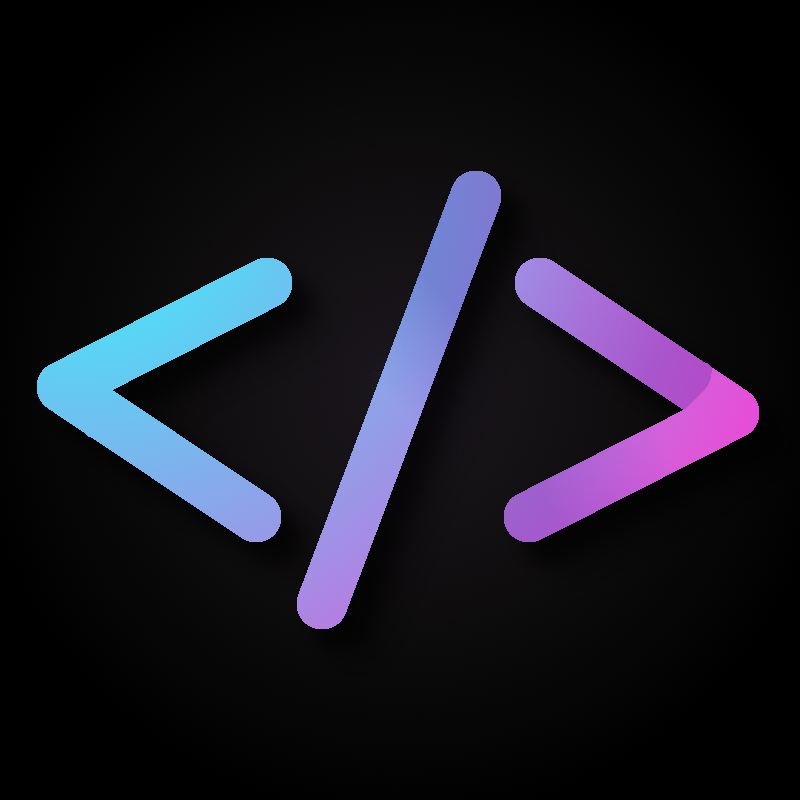
Yeah, computer science is the more about theoretical side of computation and the analysis of algorithms. For example, proving that a certain algorithm is a solution to a problem and has a particular time complexity. That’s more mathematics than practical programming.
Writing maintainable code is an art form. Like most art forms it can mostly only be learned by practice. So if you don’t have much experience maintaining long lived systems, it’s difficult to know what works and what doesn’t. Most universities don’t teach this as well, so it’s mostly something people learn in the industry.
Then I believe there’s also some aspect of pride in writing overly complicated code. It’s the belief that ”other people can’t comprehend my code because they’re not as smart as me”, when it’s actually ”I suck at writing comprehensible code”.