
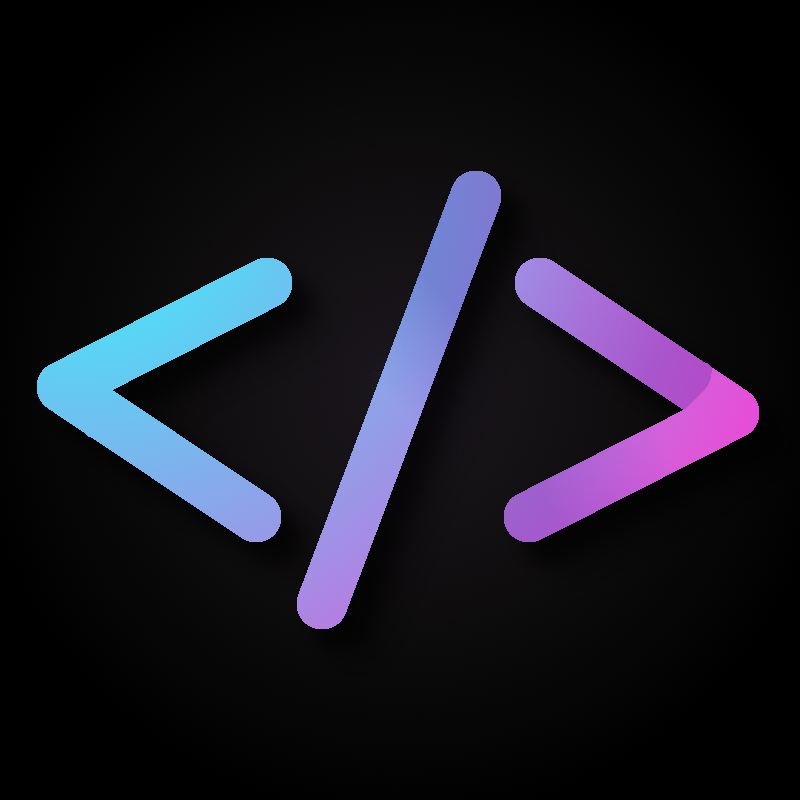
Ill try it out. I put psm 11 at one point, but got 000000 back out unless my camera was just in the perfect spot. Then i would get around 1/2 the numbers right.
Will talk about Linux, plants, space, retro games, and anything else I find interesting.
Ill try it out. I put psm 11 at one point, but got 000000 back out unless my camera was just in the perfect spot. Then i would get around 1/2 the numbers right.
the easyocr version somewhat works…but still having issues when its actually outside. Thought I would ask if anyone has figured out this issue before. Ill keep hacking away at it, but thought I would ask if the API is viable.
import cv2
import easyocr
import numpy as np
from PIL import Image
from collections import Counter
# Initialize the EasyOCR reader
reader = easyocr.Reader(['en'])
def preprocess_image(image):
# Convert to PIL image for EasyOCR processing
return Image.fromarray(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
def recognize_text(image):
processed_image = preprocess_image(image)
results = reader.readtext(np.array(processed_image), allowlist='0123456789')
# Concatenate all recognized text results
recognized_text = ''.join(result[1] for result in results)
return recognized_text
def format_number(text, length=6):
# Remove non-numeric characters and pad with zeros if necessary
formatted = ''.join(filter(str.isdigit, text))
return formatted.zfill(length)[-length:]
def most_common_number(numbers):
# Find the most common number from the list of numbers
counter = Counter(numbers)
most_common = counter.most_common(1)
return most_common[0][0] if most_common else ''
def main():
cap = cv2.VideoCapture(2)
if not cap.isOpened():
print("Error: Could not open webcam.")
return
print("Press 'q' to quit.")
frame_count = 0
text_history = []
while True:
ret, frame = cap.read()
if not ret:
print("Error: Failed to capture image.")
break
# Recognize text from the current frame
recognized_text = recognize_text(frame)
formatted_number = format_number(recognized_text)
# Update the history with the latest recognized number
text_history.append(formatted_number)
# Keep only the last 10 frames
if len(text_history) > 20:
text_history.pop(0)
# Determine the most common number from the history
most_common = most_common_number(text_history)
print(f"Most common number from last 10 frames: {most_common}")
# if cv2.waitKey(1) & 0xFF == ord('q'):
# break
cap.release()
if __name__ == "__main__":
main()
No idea. I would assume it’s the same as all other activityhub providers.
It took a bit but legitimate repo owners are starting to come over to codeberg and other alternative git sites. If we can get federation working it will be even better.
My GitHub account is getting swamped with AI created accounts following my account because it makes them look legit. It’s getting pretty bad…
Yep lots of these are popping up today. Wonder if google is going to start going hard on this.
I made good money on EDI.
I liked laravel. It’s very rails like.
True most COBOL is in person only. At least from what Ive seen. Big detriment but most systems are on physical computers…so gl!
Especially for senior Devs. It’s been pretty good eating last year or so. AI means a lot of mediocre code that needs experienced devs to de-turdify projects that are successful on paper but we’re not built of anything other than a couple of users. Fun times.
And most places are looking to get the process out of COBOL. So essentially you putting yourself out of business, slowly.
COBOL also can work with all modern day components. Like odbc/SQL etc…
Yep I worked in insurance and COBOL. Mostly getting it out of COBOL. Working remote is so nice! I’m hybrid right now it’s excellent.
I learned on gnucobol. I believe it was updated to opencobol at some point.
As someone who has worked with it, it’s not too bad. Lots of $$ if you know someone.
The biggest issue (that she goes into), is the lack of context. Why is the thing doing what it is doing is the hardest part
Same. Ive had to create a couple of custom API wrappers and each one is significantly different especially with oauth. But it almost always comes down to getting a temp token, do a thing for a time, get another temp token, so on so forth.
Unless its for myself or my family I ant gonna do it :D
I think in retirement, my goal will be to step back from the keyboard and go fishing/gardening or something. Unless I get the itch to do coding, Im not going to do it.
Sometimes ill do TDD, sometimes ill do the opposite. When I know what something looks like or should function. Im not sure what the technical term of itis, but ive heard someone call it Scaffold testing. Its making sure all the parts work as expected (Unit and integration/e2e) for your future sanity.
TDD lets you experiment and makes multiple potential solutions to a general problem. IE starting with the end first. Scaffolding lets you create a scaffold around what you already built so its more rigid. Both have their place.
Heh yeah. I feel that. Close to 20 now and Im starting to feel the churn. But its still a good feeling to give direction and see people grow. I used to be a team lead in addition to a senior dev…now im just a dev (being an individual contributor is fun again) and the 40 tabs bit resonates with me. I find that AI is good a surface level assignments like build basic CRUD/models…but it Fd up so hard sometimes its hard to come back from. Definition of spaghetti sometimes haha. I just go back to the old stuff that I know works.
I’ve heard of the term “expert beginners”.